Slingshottr: A Write-Up
Preface
I am incredibly proud of what I’ve managed to achieve with Slingshottr. It’s by no means perfect, there’s plenty I want to improve and work on, but nonetheless I never thought I’d successfully undertake such a big project by myself. Below I have written about and analysed some key and interesting elements of development, along with my thought processes and the impacts of my decisions.
Though I recommend reading from start to finish, feel free to click on any heading in the contents section that interests you!
Spotted a typo or got a question? Email [email protected].
No A.I. tools were used to create Slingshottr, nor were any used to write this post.
This post is not yet complete!
Contents
- Overview
- Physics
- World Generation
- Scrap
- Enemies
- Multiplayer
- Clara
- Shop & Upgrades
- Random Events
- Ranks, XP, & Unlockables
- Ship Types
- Settings & Player Stats
- Art
- Audio
- Playtesting
Overview
Introduction
I worked on Slingshottr for about a year before going into early-access on Steam. It was my first project in the Godot Engine, which I moved to when Unity announced runtime fees. Other than a handful of sound effects and the voice of Clara (the very talented Elizabeth Plant), I made everything myself from scratch.
Similarly, all code is my own, aside from using GodotSteam libraries to merge Godot’s multiplayer synchronizers and spawners with Steam’s networking and lobbies.
As a solo developer, I had to try to be realistic about what was achievable and stay within a reasonable scope.
The following is a non-exhaustive dive into some of the processes, challenges, solutions, and musings of this development journey. The topics are roughly organised into subheadings, but of course there is overlap and a bit of to-and-fro in terms of timeline. Enjoy! ๐
Tools
Here’s just a quick list of tools that I used in this project as I’ll be mentioning them later on.
Gameplay
As you might be reading this with no idea what the game is about, I’ve added this section to explain most of the core mechanics and features.
You are a space scrapper who has been assigned to Station 32 working under Clara. You travel from sector to sector collecting different types of scrap using your tether, either alone or multiplayer with up to 4 friends. There are enemies to avoid such as pirates, and hazards to navigate such as asteroids and black holes. There are also random events, some of which are dangerous. There is a repair station to repair your ship at, and you can tow other players if they are too damaged to get there themselves. Multiple players can tether one object to speed up the transporting process.
In each sector, the ‘scrap quota’ players must hit increases, as does the rate that enemies spawn.
There are different biomes to explore, ships to unlock, and upgrades to purchase from the merchant station.
Multiplayer in Slingshottr involves one player hosting and having authority over most objects in the game. Other players connect as clients. Anywhere I mention ‘server’, I am also referring to the hosting player, as they are both the server and a client.
Physics
It makes the most sense to start off discussing physics, as it’s pretty much the most important aspect of Slingshottr.
There are 3 main intertwined elements here:
- Player Ship Movement
- Gravity
- The Tether
Player Ship Movement
When I started the project, I started testing out ship movement and gravity by having an empty map with just a Moon to navigate around.
At this point, player movement was by impulse; players could click to fire their ship in a short burst in the direction of their cursor.
Later, following much playtesting and feedback from testers, I ended up changing player movement to be continuous. Instead of clicking giving singular impulses, players could hold the mouse down for continual movement. To further improve the experience, I created a gentle thruster sound effect (filtered white noise), alongside some particle effects.
I also spent quite a while trying out different ways of having the camera follow the player’s ship. I started with just having the camera’s position locked to that of the player, but as you can see in this video it was interesting to have it follow smoothly. Ultimately I chose the ‘locked’ camera option as playtesters found it easier to keep track of their ship.
Networking Player Movement (and more!)
Initially, I networked player movement simply by using a MultiplayerSynchronizer node to sync positions and rotations of ships. Though this worked, it was, as you can imagine, jittery. This jitter is due to not having any sort of smoothing. (Simply put the nature of networking means we can only send a limited amount of data, and data can be lost in transit.)
I realised this would be an issue for all RigidBody2D objects I would want to add, such as asteroids, enemies, and scrap.
My solution was to create my own NetworkedRigidBody2D class, which inherits from RigidBody2D.
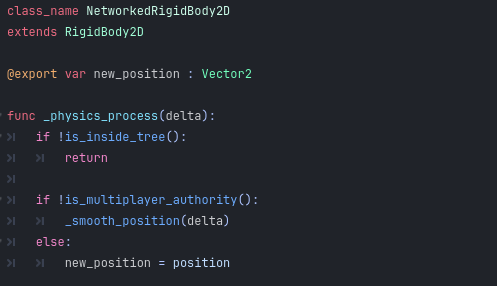
My NetworkedRigidBody2D adds a variable ‘new_position’, which is exposed to the MultiplayerSynchronizer.
Every physics frame:
- If this code is running on the server:
- Set new_position to the current position
- Else (if it’s running on a client)
- Set object’s current position to an interpolated position between current position and new_position.
I create the interpolated position in a method called _smooth_position.
I modify the position
by time (_delta
) multiplied by separate distance-dependent factor
.
position = position.move_toward(new_position, _delta * factor)
Is this enough to create a smooth experience? For most games I’d say no.
“Multiplayer is smooth as butter”
From a review of Slingshottr on Steam
However, since implementing this basic interpolation I’ve had no complaints from playtesters or players. More could be done however, a good example I’ve considered adding is client-side prediction.
The Gravity Bug
A hurdle soon appeared as I was testing. After adding gravitational fields to the game, (using Godot’s Area2D Gravity parameter) I found a troublesome bug. If a player died while under a field’s influence, then upon respawning they would still suffer a constant pull in the direction of the field, even if outside its actual range.
After respawning, players still suffered phantom gravitational pull.
Initially I thought this was an issue somewhere within my code, but since I was using the Gravity feature of an Area2D to achieve this, I considered that it could be a bug in the engine. Following some searching online, I found this to be the case. Thankfully, a pull request (82961) had been opened to fix the issue.
Unfortunately, it took longer than initially expected for this to make its way into the official releases. I decided to compile the engine myself, adding this fix. Although this worked, I could not get the (then) current version of GodotSteam to play ball with this custom compile. So, I ended up grabbing the first non-stable version of Godot that included the fix. This ended up being Godot 4.3dev2. Thankfully, this worked with GodotSteam and didn’t seem to add any new bugs! Soon after this, I added the tether.
The Tether
Up until this point players (still with just impulse-based ship movement), would collect scrap by colliding with it, then deposit by pressing spacebar whilst near the station. I had been playing some Just Cause 3 at the time, and pondered creating a grapple/tether for scrap collection.
I started off by looking through the available physics joints in Godot:
- PinJoint2D
- DampedSpringJoint2D
- GrooveJoint2D
My initial thoughts after reading about the joints was that I could probably combine a PinJoint and GrooveJoint in order to achieve the tether behaviour I was looking for, that desired behaviour being pretty much the same as a rope. The PinJoint would allow 360 degrees of rotation, while the GrooveJoint would slide in a manner preventing the tether from becoming too short or too long.
I also decided a Line2D would be a good starting point for visually displaying the tether to the player. Initially I just used a grey colour, but later created a repeating rope texture for this.
I began testing this in single player, and pretty quickly began testing in multiplayer with friends. Although it ‘worked’, it created incredibly unpredictable behaviour.
This was markedly more noticeable when players tethered to each other.
I decided to scale back, and try achieving the desired behaviour with a single PinJoint. I felt this would still work since the PinJoint has a ‘softness‘ parameter, which in practice affects how long the tether can be.
There are a lot of other factors that have gone into balancing the tether, such as mass of scrap items, and speeds and masses of players. A lot can also be said about the process of making this tether system work in multiplayer, and that can be found in the Networking The Tether section as I don’t want to repeat myself here!
The end result of all this was that players could now right-click on scrap to attach their tether to it, and it would then be pulled behind them as they flew around. Adding a bit of code to the station, the scrap would now automatically deposit when it touched the station’s forcefield.
This is of course a major oversimplification. In reality upon touching the forcefield, calls have to be made for all players who were tethered to untether the scrap object, and an RPC (remote procedure call) has to be made to the server to destroy the scrap object. Similarly, the value of the scrap has to be recorded, and more! (Which I’ll cover in the following sections.)
Networking The Tether
My initial thoughts – mistakenly – were that I’d need to replicate the physical joints of the tether of each player for each other player. I.e. when player 1 tethers something, that action needs to be replicated for all clients.
While prototyping I quickly realised this was not the case. Given the nature of the server-authoritative model, (relevant here in the server handling the positions of scrap), I actually would only need to replicate the tether joint of each player on the server.
In the end I achieved this by syncing the node_b joint variable using an RPC. This would result in the following:
- Player 1 tethers an object
- RPC is sent to the host/server to update its copy of Player 1’s grapple joint’s node_b variable.
- Host/Server updates the node_b variable.
- RPC is sent to the host/server to update its copy of Player 1’s grapple joint’s node_b variable.
- Player 1 then moves, pulling on the tether joint
- Host/Server received a new position for Player 1’s ship
- Host/Server simulates how the joint (and tethered object) is affected
- Tethered object moves appropriately in Host/Server’s game.
- Host/Server sends tethered object’s new position to Player 1
- Player 1 sees the tethered object pulled toward them by their tether.
World Generation
World generation started off very simple in Slingshottr. When the player started the game, we would instantiate a space texture (infinite tiling texture I made in GIMP), along with the player’s ship. Soon I added other prefabs to be spawned, such as a station, asteroids, and scrap crates to collect.
Quota
Very early on I began experimenting with how I wanted the scrap item quota to increase per sector. I prototyped this in an OpenOffice Calc spreadsheet, and ended up settling on the following formula.
Quota = 4 + sector2 / 2
The result is then rounded up to the nearest integer.
Example: Sector 1
Quota = 4 + 12 / 2
Quota = 4 + 0.5
Quota = 4.5
Quota = 5
Example: Sector 25
Quota = 4 + 252 / 2
Quota = 4 + 312.5
Quota = 316.5
Quota = 317
The reason the quota starts with the constant of 4 is so that the numbers aren’t stupidly low in the early sectors – I didn’t want a sector to be cleared with just one piece of scrap.
Procedural Generation
World Setup
When the game starts, the background space texture is spawned in with a random rotation. This is enough to give the effect of a different area of space in each sector.
Initially the repeating background texture was the only background feature in every sector. However I felt more could be done to make things more immersive in this regard, so I created a few singular star textures that could be randomly placed in the background. The positions of these textures (and all spawned objects) was in part controlled by a seed for pseudorandom number generation.
Parallax
I needed to create a system for handling the effect of parallax – I felt it looked wrong having stars in the background moving across the screen at the same rate as foreground objects.
Also I was somewhat inspired by the parallaxed backgrounds in games like Terraria.
The subtle difference between no parallax and the final parallax.
To create this system, I made 3 dictionaries to hold all the foreground, middle ground, and background sprites. (Only for sprites unimportant to gameplay.)
Weird parallax effects near edges of the map.
I would then run through each sprite in the array and adjust its position relative to the player whenever the player moved. This created the effect of parallax. However, this all became weird near the edges of the map, as I have the camera confined within the playable space.
So I had to add in some effective ‘dead zones’ where parallax would stop being applied (or more accurately, only be applied in the axis that the camera remains unconfined in.)
This meant that, at the far sides of the playable area, parallax could still happen up and down, whereas at the top and bottom of the playable area, parallax could only happen left and right. Meanwhile at the corners, no parallax would occur.
After fixing the weird parallax effects near map edges.
Seeds & Pseudorandom Number Generation
For all objects in the game (except players, enemies, and scrap), I wanted their positions to be the same for every player. This could theoretically be done by networking every object to sync positions and rotations, however the amount of bandwidth this would require is ridiculous.
So the solution I settled on was to sync a seed value via RPC when a new sector is generated. Clients would then use this seed value to spawn in all the objects onto the map. But how would the positions be correct? Well by inputting that seed value into the same pseudorandom number generator, we can just request values from the PRNG and get the same numbers.
So a simplified example of how background objects are initially positioned:
@rpc("reliable", "call_local")
func _set_object_position_using_seed
(_seed):
var rng =
RandomNumberGenerator
.new()
rng.seed = _seed
new_object.position = Vector2(rng.randi_range(-100, 100), rng.randi_range(-100, 100))
The above code would result in every client setting the new_object to the same position. Say the seed for the sector was 12345. Here’s what would happen:
- The server would call
_set_object_position_using_seed.rpc
(12345):
- Every client would receive the RPC, and run
locally._set_object_position_using_seed
(12345)
- In doing so, each client would:
- Create a new
RandomNumberGenerator
. - Set the
seed
of theRandomNumberGenerator
to 12345. - In setting
new_object.position
,rng.randi_range(-100, 100)
is called.- The
RandomNumberGenerator
returns a value between -100 and 100 based upon the seed
- The
- Create a new
- Each client would set
new_object
‘sposition
to the same point. E.g. (-35, 50).
And voila. Of course this is just an example, you wouldn’t use a method like this to set every objects position as you’d be needlessly RPCing the same seed over and over.
Instead we send the seed over once for the new sector, then the clients set up their number generator with that seed, and handle running through every object they need to spawn and setting positions purely with their number generator.
Biomes
Inspired by games such as Minecraft, Terraria, and Valheim, I’ve always wanted to included different biomes into a game I’ve made. I feel it can add so much – you’ve been exploring one type of area and get used to its conventions, such as types of flora and fauna – suddenly you find yourself somewhere very different, unsure (and excited to explore) what might be around the corner.
Since Slingshottr is sector/round-based with a very limited map size, I didn’t need to worry about how I’d handle boundaries between biomes or anything of a similar nature. All I needed to do was have a way of selecting a biome, informing clients, and then generating the world based on a modified ruleset.
The ruleset so far for the basic type of sector was very simple. Objects and scrap would effectively be spawned in totally random positions. The main exception being that I prevented certain objects from being spawned in certain areas.
For example, a black hole should not be allowed to spawn on or beside the station. In this instance, since I always have the station at ( 0, 0 ), it’s as simple as disallowing a black hole from spawning within the coordinates ( -500, -500 ) through ( 500, 500 ). This ensures no black hole spawns within a 1000×1000 square of the station
So with the introduction of biomes, stored simply as an enum, I decided things would work in the following way.
- New sector is entered
- Server picks biome
- If round (sector) number <= 1, NORMAL biome
- Otherwise, 50% chance NORMAL biome, 25% ASTEROID_FIELD, 25% AMETHYST_NEBULA
- Server RPCs biome to clients
- Clients spawn non-networked objects based on given biomes ruleset
In the instance of entering an Amethyst Nebula, the server will spawn networked objects such as scrap and Amethyst Crabs, while clients handle background nebulae, stars, crystals etc.
Scrap
Scrap collection and depositing started off very simple. As previously mentioned, players would bump into scrap to grab it, then press spacebar by the station to deposit it.
In every sector, the quota – how much scrap value must be collected – increases. At this point in development the only scrap item was a crate, so the ‘value’ of a scrap item was always 1. If the quota were 5, players would need to collect 5 crates.
I wanted to have many different types of scrap with different values, so as not to have things be too repetitive. It made sense to me that as you reach higher sector numbers the scrap spawning should be of higher value (since quota also increases each sector).
I also thought it’d be interesting to the gameplay to have some scrap that is dangerous to handle.
I wrote code to handle always spawning 20 items of scrap, in a manner whereby we only spawn the lowest value scrap that 20 pieces of would still hit quota, or higher. So the limit for lowest value of spawnable scrap is quota / 20.
Similarly, I didn’t want the sector to be completed using 1 piece of scrap, so I limited the maximum value of spawnable scrap to quota / 2.
Before writing this code, I once again mocked up what that would look like – in terms of which sectors certain scrap would or wouldn’t spawn in – using OpenOffice Calc and Obsidian.
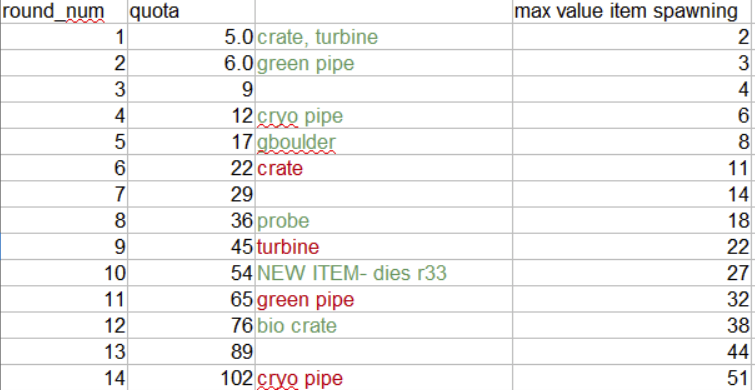
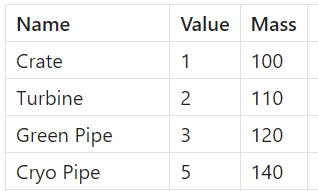
In the above, the green text denotes scrap items that begin appearing at that sector (round_num), whilst the red text denotes the sector when the scrap item no longer spawns (due to its value being too low).
Since I allowed scrap to be destroyed by black holes and sentry asteroids, I also wrote some code to check if the sector’s quota can still be hit. If it cannot, we move to the next sector as if the player had completed it. I debated instead spawning replacement scrap, but in the end I felt that purely moving to the next sector felt the least intrusive into gameplay. Also this situation is rare – it has never naturally occurred across the thousands of sectors I’ve played.
Networking Scrap
I use the previously touched upon NetworkedRigidbody2D class I made as the base for all scrap (I made a scrap class that inherits from my NetworkedRigidbody2D class). Along with a MultiplayerSynchronizer, this handles smoothly syncing scrap positions for all players.
There have been tricky elements to work out however. For example, upon spawning, I had to make the scrap do the following for non-host clients:
- Set freeze = true
- Set freeze_mode = RigidBody2D.FREEZE_MODE_KINEMATIC
- This type of freezing would allow the scrap to be moved via code if necessary, but not moved client-side by clients tethering. This was important else scrap positions would jitter due to the physics simulation on client and server being in disagreement.
- Set gravity_scale = 0
- This would prevent scrap fighting with gravity for clients.
Wrecks
I added wrecks upon the suggestion of a playtester. The tester had suggested giant, enterable abandoned ships that you could then disassemble for scrap. I loved this idea, so started prototyping it by creating a relatively small ship to be pulled apart. I still want to take this idea to its full extent.
Along the same vein, I also experimented with adding other objects that could contain scrap. For example I added a giant asteroid that players could fly their ships into – this asteroid would contain a piece of very high-value scrap such as an Iridium Bolt.
Enemies
Pirate
Pirates were the first enemy I designed for Slingshottr. I was partially inspired by the style of naval ships in space found in Treasure Planet: Battle at Procyon (2002). Instead of an old naval design, I chose a viking aesthetic.
Since this was my first attempt at an NPC (non-player-character) in Godot, I decided to keep their behaviour very simple. They would spawn just outside the sector and make their way in, targetting players and passive NPCs. This targetting would involve sounding their horn, speeding up, and ramming the target until it is destroyed.
Originally pirates were effectively immune to damage, and could only be destroyed by ‘baiting’ them into a black hole. I added a drone upgrade that allowed the player to kill pirates, but players would generally not acquire this upgrade very early on. As a result I decided to make it so that each time a pirate rammed a player, the pirate would also take damage. This way they would not be a nightmare to deal with in the early game.
Ship Cutter
The ship cutters were inspired primarily by the spider-like droids in the Star Wars prequels, seen taking apart Obi-Wan Kenobi’s ship.
I made these nanobots ignore collisions and gravity. They would move in erratic straight lines. I decided they should only target the player if they were very close, latching on until they are shaken off, or the player dies.
With slower ships it would not be possible to shake them off, so I decided the repair station could double as an antidote to the ship cutters. If ship cutters enter the repair station’s forcefield, they are destroyed.
This makes a mad rush for the player when they are latched onto; the player must forget whatever they were doing and try to get to the repair station as quickly as possible. Similarly, this may involve other players towing them to get them there faster.
Amethyst Crab
The Amethyst Crab is the first boss-type enemy I’ve added to Slingshottr. It’s biome-specific, so players need not worry about it for the most part.
I designed it’s behaviour as follows:
- If the player enters a distant target range, the crab will ‘wind-up’ preparing to jump.
- If the player remains in this range, the crab will then jump, darting towards the player.
- If the player is within a closer range however, the crab will scuttle after them.
The crab is immune to drone fire and collision damage, and no other hazards spawn in the Amethyst Nebula biome that it is found in.
I thought it would be awesome to have the tether come into play here, so I decided that the only way to defeat the crab would be for players to tether and pull off both its legs. After the first leg is removed, the crab’s speed is reduced.
I also used sound effects to highlight what the crab is about to do, such as the ‘wind-up’ and jump sounds, as well as a sound for when it begins targetting the player. Similarly, if the player is in range, the crab’s eyes will follow it.
Finite State Machine
Though all the enemies effectively use some form of finite state machine, I made a much more clearly defined one for the crab. I settled on 3 states – IDLE, CHASING, and JUMPING.
- The IDLE state would be when there are no players in range, the crab would just sit there looking around and occasionally blinking
- The CHASING state would occur once a player has been detected (and is within a minimum distance), and would involve the crab scuttling in the direction of the targetted player.
- The JUMPING state would occur when a player has been detected but is not within CHASING distance. The crab would get ready to jump, and then jump in the direction of the targetted player.
- If the crab is in a non-IDLE state, and its target is lost, it should return to the IDLE state.
I wanted the crab’s eyes to track the player, and at other times for it to look around randomly and blink.
I used a Timer to handle the 3-8 second wait between blinks. Upon the Timer ‘timeout’, I check if the crab’s pupils are visible. If they are, we hide them, change the colour of the rest of the eye to look like an eyelid, and set the Timer to 0.1s.
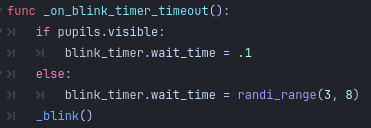
Then, upon the subsequent ‘timeout’ 0.1s later, we reshow the pupils, reset the eye colour, and reset the Timer randomly between 3-8s. If the crab has no target, we also randomly offset its pupils.
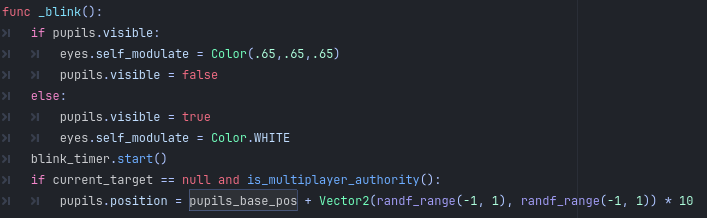
Multiplayer
I started making Slingshottr multiplayer using Godot’s inbuilt networking, based upon ENet. This would allow players to connect to eachother via IP address, but would require port forwarding or some similar method of allowing connection.
For early playtests this was no issue as I could just port forward on my router, but obviously it wouldn’t be sustainable as at some point a player other than myself might want to host!
The question that arose was how do I want to handle the issue of establishing a connection?
I researched the options, and looked at how existing games handled it.
I knew Steam was an option from the beginning; the only negatives I could think of were a) multiplayer wouldn’t work if Steam were down, and b) I didn’t know whether Godot had any support for Steam APIs.
Some games, such as Among Us, seem to use a system where clients’ games contact an intermediary server which establishes the connection for them (hole punching). I considered this option, but felt having to maintain my own server(s) for this purpose wasn’t a route I wanted to go down.
After discovering the GodotSteam and the associated SteamMultiplayerPeer project – and seeing that other Godot game developers had successfully used these tools to achieve seamless multiplayer experiences – I decided that I would rely on Steam for establishing connections.
It didn’t take too long to modify my existing networking code to utilise Steam’s multiplayer features – SteamMultiplayerPeer acted effectively as a drop-in replacement variable for most things. Despite this, for simplicity of scope I chose not to maintain the option of direct connection via IP. This is always something I could revisit however.
Managing Bandwidth Usage
During the first test since integrating with Steam’s multiplayer, I found that clients would at some point begin to lose sync with the server. This sometimes occurred straight after joining, and sometimes after a minute or two of play time.
Reading through the console logs (and searching online) it became apparent that Steam was rate-limiting the connection. So my issue was Slingshottr was using too much bandwidth. This had not been an issue up to this point as (assuming players had a fast connection) there had been nothing to rate-limit the game.
I immediately began jotting down ideas for reducing bandwidth usage in my notebook.
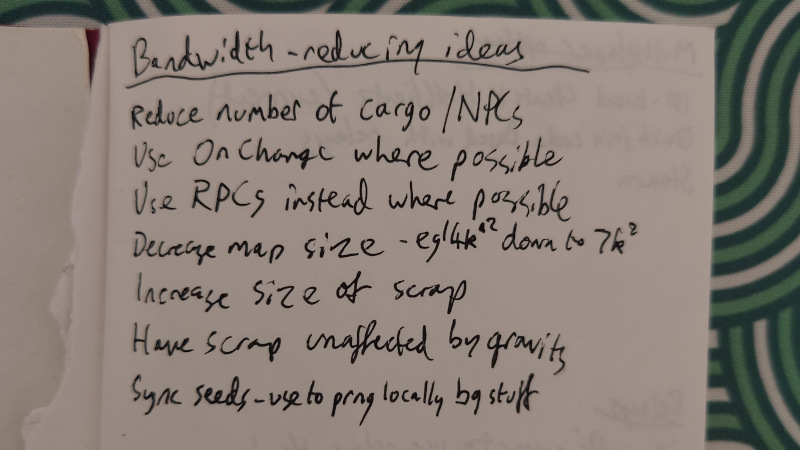
Let’s dig into my initial ideas here.
- “Reduce number of cargo / NPCs.”
- By literally spawning fewer scrap items and NPCs, we’d be syncing fewer positions, rotations etc. every network frame.
- “Use On Change where possible.”
- Godot’s MultiplayerSynchronizers allow each synced parameter to be synced Always, On Change, or Never.
- By switching parameters from Always to On Change we’d reduce bandwidth usage as we’d no longer be syncing that parameter’s value every frame.
- “Use RPCs instead where possible.”
- Using RPCs would make it far easier to see when and where data is being synched and have more granular control of bandwidth use.
- However, for many things it could be less maintainable, as I’d be writing a lot more code.
- “Decrease map size – e.g. 14k2 down to 7k2.”
- This idea in and of itself would not reduce bandwidth use. However, by reducing the total playable area of each sector, we could spawn fewer objects (reducing bandwidth) without making it feel more empty.
- “Increase size of scrap.”
- Similar to the above point, this wouldn’t directly reduce bandwidth usage, but it would allow us to have fewer objects (reducing bandwidth) without increasing emptiness.
- “Have scrap unaffected by gravity.”
- This is an interesting one. My thoughts here based upon the fact that when scrap is spawned, it isn’t moving. Combine this fact with point 2. (using On Change for synching position/rotation) and we’d find virtually no bandwidth use.
- But as soon as scrap enters a gravitational field (or the player tethers it) it would move and start syncing data every frame. If we removed the effect of gravitational fields, scrap would not move unless collided with (rarer) or tethered by the player, thus potentially saving a lot of bandwidth.
- “Sync seeds – use to prng locally bg stuff.”
- Up until this point, I had been having the few background objects that existed, (“bg stuff”), be created by the server and positions & rotations synced across the network.
- By instead having these objects (that were unimportant to gameplay and non-interactable) created by each client, there would be no need for all the excess data being synced.
- If you’ve already read the Seeds & Pseudorandom Number Generation section, you’ll know I did indeed implement this change.
I ended up implementing points 1, 2, 3, 4, and 7, and this reduced bandwidth usage more than enough. I was happy not to need to implement points 5 and 6 as I felt they would have the highest negative impact on gameplay.
Bugs
One of the most persistent bugs I encountered in this entire project involved the behaviour of tethers over the network. Simply put, non-hosts would find scrap harder to pull than the host would. This is because when a non-host player tethers an item, the actual joint connections are made on the server. I had to make the joint connections this way since the server has authority over scrap, so without doing this the scrap would not move when tethered by a client.
This introduces a small element of delay – when the player then moves, their new position is sent to the server, where it pulls on the joint, pulling the scrap. The scrap’s new position is then sent back to the player. This delay effectively makes it feel to the player like the scrap is far heavier than it should be.
This issue is exacerbated by the linear interpolation I perform (for smoothing purposes) on the positions of the scrap and other players.
I looked at many potential solutions to this issue, and tested several. One of the easiest to implement that I tested was making it so that when a player tethers scrap, the scrap’s network ownership is transferred to that player. The immediate reason for not using this fix is that I allow multiple players to tether one item. This is something I wasn’t willing to get rid of as I feel it’s a key feature for teamwork.
In the end my solution was somewhat (very) hacky, but has worked well enough for now that no player has noticed and complained. The solution I came up with was to boost player speed (relative to their chosen ship) when tethered (if they are not the host). It’s not clever, it’s not pretty, and I’m sure there will be situations where it may not be ideal, but for now it works.
Clara
Overview
My plan for Clara, (the player’s line manager operating the space station), was to have a bubbly personality who would both randomly interject with useless or comedic comments, but also provide actual informational value. This value would come from things like warning players of incoming enemies, dangerous events, and letting them know how close they were getting to their quota for the sector.
Writing & Voice Acting Workflow
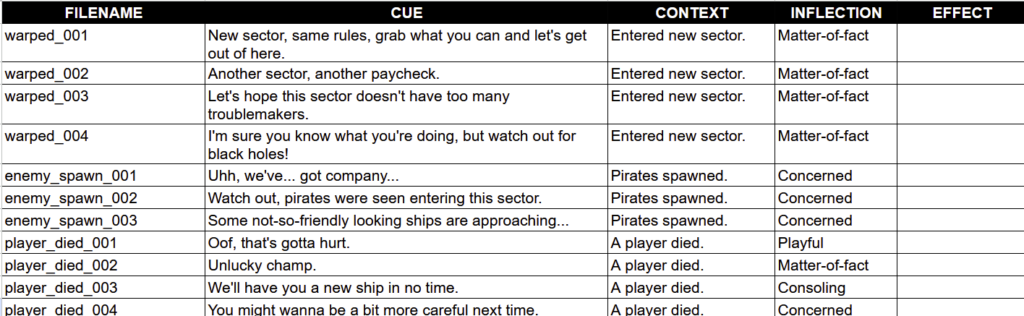
The workflow I decided on for writing and recording voice acting was fairly straight forward. After some research on industry standards, I ended up creating a spreadsheet in OpenOffice Calc (later Google Sheets). In this spreadsheet I had 4 main columns as shown in the image above.
‘FILENAME‘ was the name of the final file I’d create when I’d cut the voice acting, aka its ‘slug’. This name would also be used for Loading & Organising. ‘CUE‘ was the actual line for the voice actress to perform. ‘CONTEXT‘ was extra information on when the line might be played, and ‘INFLECTION‘ suggests to the voice actress the general tone of voice to use.
‘EFFECT‘ was only used for one or two lines, wherein I’d request a certain non-verbal sound during the line. For example, Clara jokingly making a radio static sound before saying “Station log: today I saw an asteroid shaped like a heart.“
I would then send a copy of this spreadsheet to the voice actress Elizabeth, along with a very basic character description.
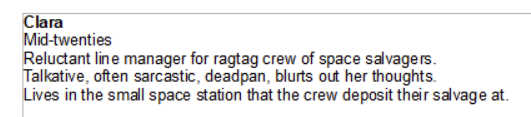
Elizabeth would then send me back a recording of all the lines with multiple different takes. Using this file and the spreadsheet, I would then (using the program Audacity) pick the best takes and cut up the lines, saving them with their correct ‘slug’ file names.
Subtitles
I added subtitles pretty soon after the first of Clara’s lines. One of the driving factors for this was reading about Ubisoft’s figures for subtitle usage.
I decided due to scope as a solo developer, I would purely subtitle Clara’s lines, not any sound effects. Similarly, I didn’t plan to add any other accessibility options (e.g. high contrast), just a simple white text with slight black border.
So how does it work? When Clara wants to say something, we check if the player has subtitles enabled, which are on by default. (This subtitle setting is handled as part of Settings & Player Stats, explained later on.) If they are enabled, we set the text property of the RichTextLabel to the relevant subtitle. Then, when the voice line finishes playing, we clear the subtitle.
Loading & Organising
I had to write a system to load and organise both the audio files of Clara’s lines, and the text for subtitles. The system would also need to be able to play lines as requested from other methods and classes.
The system I created worked in a couple of steps.
- First, it opens a file containing all subtitles. This file is formatted similarly to a CSV, it provides the subtitle, and the slug for the line.
- Next, it builds an object. My AnnouncerClip object contains the subtitle string, and the relevant AudioStream (the voice line), which is found using the slug.
- Finally, again using the slug, it places this object into the correct array.
This system, though obviously limited (e.g. can only have each line in one array, can only have one array per slug, a typo in the CSV can break everything, etc.), works well for this project. It also required very little code to implement.
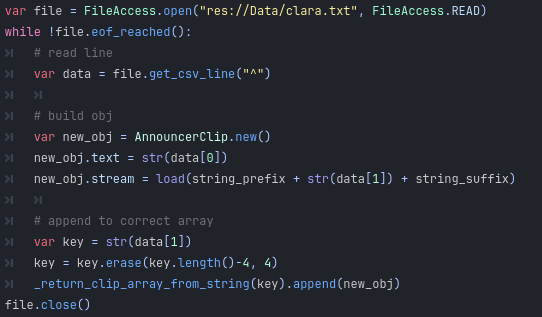
string_prefix and string_suffix are just the location of Clara’s voice clips and “.mp3” respectively.
The Announcer System
There are two main ways Clara can end up speaking a line of dialogue. One is a timer-based loop I made so that every now and then she randomly says one of her miscellaneous (i.e. not related to gameplay) lines, and the other way is having a specific type of line called for by another method somewhere.
The method that accepts this type of call requires a parameter for priority. I added this priority parameter as I felt that it was important for gameplay-relevant dialogue to be prioritised over general chatter. For example if Clara is mid-sentence rambling about her new trainers, and a pirate enters the area, she should immediately cease talking about her trainers and tell the player about the presence of a hostile.
I also didn’t want to have a situation where Clara keeps repeating the same dialogue over and over, as it could really ruin the player’s immersion. So to combat this, I added an array that would keep track of recently spoken lines, and if Clara tried to say one it would prevent it. These recently spoken lines would be cleared from the array after a period of time.
Similarly, I added percentages to control the chance of Clara speaking a line under certain circumstances. So for example she will comment on a player’s death 75% of the time, but only comment on entering a new sector 50% of the time. Again, the aforementioned recently spoken lines array can override this. The reason for these percentages are simply that, with the example of commenting on a player’s death, if 3 players die in a row it is unnecessary and a bit repetitive for her to comment on all of them.
Walkie-talkie Sounds
Although a bit illogical when you think about it, I thought it would be fun to have all Clara’s lines preceded and followed by a walkie-talkie sound. Obviously it would be silly to manually stitch these sounds on every line. Similarly, if Clara was speaking, and immediately started another line, I didn’t want the walkie-talkie sound to repeat.
I achieved the desired behaviour by simply checking if there were no queued lines. (When we want Clara to say something, we add it to a queue.) So, if Clara is speaking, and finishes a line with no queued lines, we play the walkie-talkie end sound. If Clara isn’t speaking, and a line is added to the queue, we play the walkie-talkie start sound.
Networking Clara
The networked aspect of Clara and her dialogue is fairly straight forward. When a request is made for a line of dialogue to happen, the host chooses the line and then RPCs that information to the clients so that they can also play the line.
Clara’s Effect on Gameplay
Even from the early implementation – when Clara only had a few lines such as welcoming the player and commenting when they died – the feedback from play testers was fantastic. They felt she added a lot to the immersive feel of the game, specifically regarding how her comments tied in with events that were happening.
I felt she served a second purpose well; she was a useful tool for explaining things to players without having to just use text prompts. For example, since adding her comments about percentage of quota complete, along with the visual lights on the station, play testers stopped complaining about not knowing how much scrap they needed to collect.
Clara’s comments have also meant players can receive new information about things happening elsewhere in the sector without needing to be there. If two players are playing at opposite ends of the sector, they will likely know if the other has died as Clara will usually say something. Similarly, players don’t need to see if enemies are entering the sector, as Clara will comment on it.
Similarly, I think Clara’s comments when events occur have helped prevent confusion. I can’t say for certain, (as I included the new Clara lines with the event patch), but play testers did not seem to have any problems understanding the random events when they occurred for them the first time.
Shop & Upgrades
I added upgrades to the game fairly early on, initially envisioning them to behave similarly to upgrades found in games like Risk of Rain – pickups that are fairly innocuous at first, but can be stacked to great effect.
I created a system that would, based on the amount of scrap value deposited, spawn a random upgrade near the station for each player.
I started with two upgrades, basically health and speed increases, but soon expanded to try out more.
Upgrade | Sprite | Effect |
---|---|---|
Booster | ![]() | 5% speed increase |
Armour Enhancement | ![]() | 25% health increase |
Grapple Extender | ![]() | 5% grapple range increase |
Luck Injector | ![]() | 2% chance to ignore all damage |
Second Wind | ![]() | 1% chance to cheat death |
Defense Drone | ![]() | deploys a circling defensive drone |
Pitstop | ![]() | 50% increased repair speed |
Scrap Magnet | ![]() | scrap is attracted to your ship |
Rocket Barrage | ![]() | (defensive) heat-seeking missiles |
I could write a lot here about the intricacies of implementing all these upgrades, and the full ins-and-outs of how they all work, but I’m aware how long this document already is!
There were of course many considerations to make regarding UI, and (long story short) I ended up deciding each upgrade should be displayed as a kind of status icon at the edge of the screen. I later added text to these icons so that the number of each type of upgrade picked up is shown. I also added text when the player hovers their mouse on the status icon to give further information.
I also created a banner that would pop up with a sound when the player collected an upgrade. This banner would display the name of the upgrade plus a description of its effects.
A problem with this system was that often during play tests, one player would swoop by the station when upgrades were dropped and take them all. This annoyed other players.
A possible solution to this would have been spawning the upgrades client-side only. This would mean each player would only see one upgrade that they could collect.
After a lot of discussion with play testers and gathering their thoughts, I decided to instead make upgrades purchasable at a shop station – no longer being given for free by the station.
The reason I chose this option was partly the aforementioned upgrade hoarding, but also play testers wanting to be able to specifically pick their upgrades to try out different ‘builds’.
So in this new system, every time any player deposited scrap, all players would receive that scrap item’s value added to their balance. Then when a player purchases an upgrade, only their balance would go down.
Random Events
I decided to add random events to Slingshottr to provide both some more interesting occurences and a way of switching up the gameplay somewhat.
Events can be purely visual – such as the Meteor Shower, non-dangerous – for example Clara’s Spacewalk, or dangerous – like the Quasar Burst.
Visual events don’t affect gameplay in any way, but will yield a comment from Clara about what is happening – Clara will always comment at the beginning of any event.
All other events serve to change the pace of the game, and the immediate goals of the players.
Event Summaries
Click on the Event names below to read a summary of what the event entails.
Clara’s Spacewalk
During this event, depositing at the station is halted while Clara carries out maintenance checks on the station. She can be seen floating around tethered to the station, and will speak aloud whilst performing her checks. A progress percentage is flashed up by the station every few seconds. Any scrap left near the station will not be deposited during this period, so it will continue to move with whatever inertia it had.
Quasar Burst
This a catastrophic event. After a few seconds of a warning tone and flashing symbol on the player’s screen, a blast of energy will rocket across the sector, damaging or destroying any player in its path, shaking the screen, and emitting a rumbling sound. The burst will always pass in a straight line through the station, but can come from any direction.
Ghost Ship
This event begins with Clara (and all sound effects) becoming muffled and echoey, along with in-game music stopping. Fog will fade in, and after a number of seconds the Ghost Ship will appear somewhere on the map. At the same time, eerie sounds start playing. The Ghost Ship will head toward the station, and remain in the vicinity for 60 seconds before disappearing. It deals massive damage to players on contact. After it disappears, the fog recedes, music returns, and Clara and all sound effects lose their muffle and echo.
Meteor Shower
This event has streaks of light sail across the sector. It’s purely a visual event.
Space Whales
This event begins with a pod of space whales spawning in the sector. They will swim across the sector from right to left before despawning. It’s purely a visual event.
Implementation
I created an Event Manager class to handle running events. Before writing any code I thought about how I wanted events to run, and what sort of variables might be required. I settled on a three-stage system.
Every event would have a pre-event stage, an event stage, and an end stage (basically 3 separate methods). My reasoning for this was as follows:
- We should be able to make things happen in the run-up to an event, e.g. warning the player
- Specific things happen during the event.
- At the end of the event, there may be things we’ve changed that we want to reset.
Here are some simplified comparisons of events and their stages.
Quasar Burst Event
Pre-Event Stage
- Set pre-event duration to 15s.
- Set event duration to 5s.
- Stop in-game music.
- Clara warns player.
- Play alarm tone & visual.
- Disable Clara.
Event Stage
- Stop alarm tone & visual.
- Spawn quasar burst.
End Stage
- Destroy quasar burst.
- Re-enable Clara.
- Start in-game music.
Meteor Shower Event
Pre-Event Stage
- Set pre-event duration to 0s.
- Set event duration to 1200s.
- Clara comments on beauty.
Event Stage
- Spawn meteor shower particle effect.
End Stage
- Destroy meteor shower.
So the full flow of the Event Manager is:
- Sector entered
- Event Manager decides if an event should occur
- Event Manager decides which event should occur
- Event Manager begins Pre-Event Stage
- Pre-Event Stage ends, Event Stage begins
- Event Stage ends, End Stage runs
- (Leaving the sector during any of these moments also triggers End Stage)
Impact on Gameplay
During Clara’s Spacewalk players cannot deposit scrap, so are forced to gently bring scrap and pile it by the station. This is in contrast to the normal tactic of flinging scrap in the station’s general direction, knowing it will deposit on contact. The Scrap Magnet upgrade is a tactical upgrade that can make dealing with this event a breeze.
During the Quasar Burst, and somewhat similarly the Ghost Ship event, player goals change from collecting scrap to trying to survive. If there are many enemies around during these events this can raise the stakes even more.
The Quasar Burst encourages a mad rush to get away from the station and (hope) not to be hit. Similarly the Ghost Ship event forces players away from the station due to the behaviour of the ghost, but this time for a longer period. Add to that the almost opaque fog drifting across the screen and this makes players less willing to zoom around the map.
Networking Events
Though a simple task on the surface, (only allowing the server to pick and run events, telling clients what to do via RPC, remembering to also send RPCs to reset changes), networking the event system yielded many bugs.
Generally these bugs were fairly easy to diagnose. For example, upon adding the Ghost Ship event and testing in multiplayer, players were finding sporadic issues such as music not returning, echo/muffle remaining, and fog not dissipating.
After some more specific testing, I found that these issues were only occurring under the following conditions:
- For clients, (not the server/host)
- When a sector is completed before the event has naturally finished.
After looking over my event code again, I found that the reason for these issues was simply that I had forgotten to add .rpc
to the ‘end event’ method calls. When the server should be telling clients to end the event because we’ve left the sector, the RPC was never being sent – because I’d forgotten to put the .rpc
at the end! Meanwhile in all other instances event RPCs were being sent fine because I’d remembered the .rpc
.
A literal example: instead of music._return_to_normal.rpc()
I had typed music._return_to_normal()
. This would only run the _return_to_normal()
on the host, not the clients. So the host would find their audio back to normal, while other players would not.
Ranks, XP, & Unlockables
It was on a long countryside walk that I happened to think of adding a rank system to Slingshottr. By no means was this an original idea – games like Lethal Company came to mind.
I pulled out my notebook and started jotting down some possible rank titles.
On the left are normal rank titles, and on the right of the page I started thinking of titles (only one is shown in the image) that I thought could perhaps be suffixes or prefixes to the main rank. My thinking was that I’d have some sort of XP system that would award new ranks at certain milestones, and then the prefix/suffix ranks would be awarded based on particular achievements.
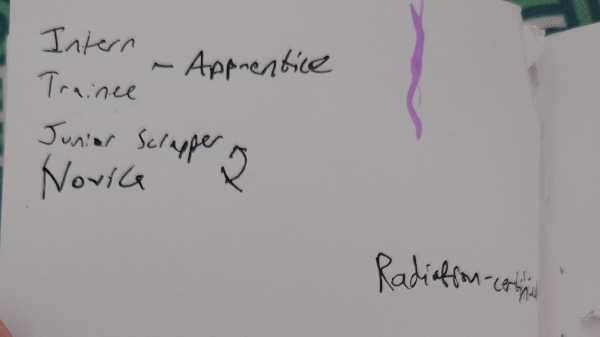
For example, Radiation-Certified was a suffix I thought that players could unlock by depositing their first Rad Crate (meaning they’d have to at least reach sector 15). I considered that this suffix rank could bestow on the player a reduction in damage taken from radiation.
I never ended up implementing suffix/prefix ranks, as on a scale of effort to impact, it was fairly low impact and medium effort. Other work took precedence.
Tied into this rank system was the ship-unlock system. Once reaching a certain rank, players gain access to a new type of ship. I ended up reusing the ‘upgrade’ UI banner for this purpose to inform the player of their new available ship.
Ship Types
I decided there should be multiple different ships the player could choose from in Slingshottr. I felt this would lend itself well to different play styles and perhaps provide greater replayability.
From the beginning I knew I wasn’t just going to have the ships look different (different sprites) – each ship would play differently. I achieved this by giving them each unique mass, velocity, and health. I also modified gravity scaling for the Barge – i.e. how affected it was by gravitational fields. The Cruiser was unique in receiving a permanent Drone upgrade.
Ship Name | Sprite | Mass | Speed Multiplier | Health | Gravity Scale |
---|---|---|---|---|---|
Lander | ![]() | 100 | 600 | 100 | 1.00 |
Barge | ![]() | 600 | 300 | 500 | 0.65 |
Lander Mk. II | ![]() | 85 | 850 | 75 | 1.00 |
Cruiser | ![]() | 400 | 600 | 300 | 1.00 |
The process of balancing these ships involved a lot of trial-and-error and playtesting. This was partly due to the physics aspect of things, but also due to other systems that came into play. For example, though a Barge may start as a very slow and lumbering ship, some players were able to make it incredibly fast by focusing on collecting Thruster upgrades. This caused a massive shift in the game’s balance.
This ‘unbalancing’ effect was massively reduced through modifying how upgrades worked – e.g. whether their effects compound, alongside switching to a shop-based system.
Settings & Player Stats
There were some basic settings I wanted to let the player modify and store. These include the volumes of the audio buses (Master, Music, Effects, Voice), and whether subtitles are enabled.
Since I was still new to Godot I looked around for what the most sensible option was. Lo and behold ConfigFile was an inbuilt class that looked great for this. Saving settings was as simple as:
var config = ConfigFile.new()
config.set_value("Audio", "music_volume", value_of_music_volume)
config.save(config_file_location)
However, I had to bear in mind that I may add new settings over time, and didn’t want to make the game crash for players when they moved from an older version (with an older config file) to a newer one that expected values that didn’t exist.
Thankfully, once again it was simple. In my loading code I used ConfigFile‘s has_section_key()
method to check if the setting I was trying to read existed before reading it.

Then when settings were saved, I would save the new section into the config file.
Player Stats
I’ve always been one to enjoy when a game provides a stats page about your playtime. Minecraft is a great example of this, showing you the number of times you performed certain actions, mobs killed, distance walked etc. I decided both for my own entertainment and that of like-minded players that I would implement some sort of statistic recording into Slingshottr.
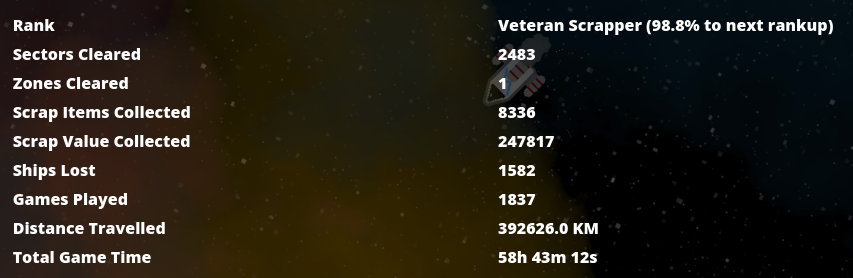
I created another ConfigFile for this purpose, and initially only tracked Sectors Cleared and Distance Flown. After I started tracking who had deposited scrap (for the in-game TAB screen), I added scrap stats to the file too.
I’ve ended up recording quite a few statistics for players to view, however there are still more granular statistics I’d like to add, such as Quasars Survived, and Pirates Killed.
Art
Of all the disciplines in game development, 2D and 3D art are probably my weakest points. In early versions of the game I went for an almost cartoonish 2D art style, similar to my 2017 game Valentelephants.
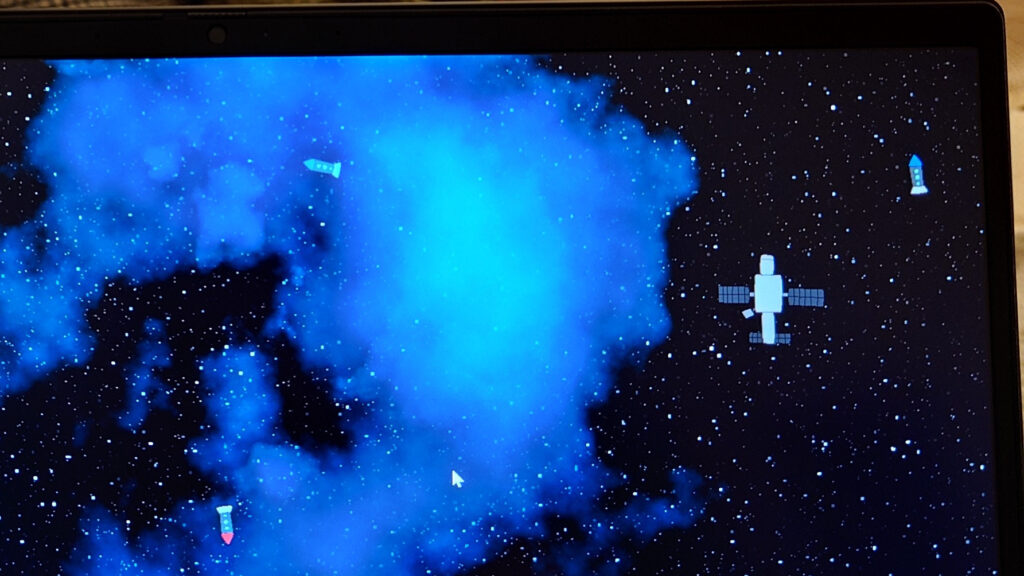
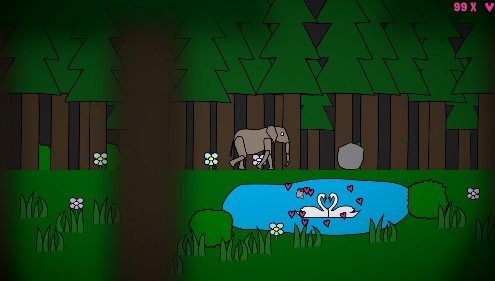
I soon realised that creating art in this style did not fit with what I was capable of creating for other elements, such as the starry background and the nebulae. The style of background and particle effects I was capable of creating was much more fitting with a pixel-art style.
As I had previously made a lot of pixel art for a project in the past, (A Bad Day at Work), I was aware of how much longer it may take to make. However, this concern was unfounded, as for A Bad Day at Work I was also creating normal maps for every asset. I was not doing so here.
So I decided that was the best option for a consistent art style. I began redrawing assets such as the station and player sprites in a pixel art style, and continued to create new sprites in this manner.
I created the starry background using noise generation in GIMP, and then adding a threshold filter. Similarly, I created the nebulae sprites using noise generation in GIMP.
In this period of time I also created a colour palette. This palette was not so much for the game as a whole, but for the nebulae that would spawn in each sector (this was before I added different biomes, which each have their own colour scheme).
Prior to this colour palette, I had settled on only using purple and blue nebulae, which looked okay but didn’t offer much variability.
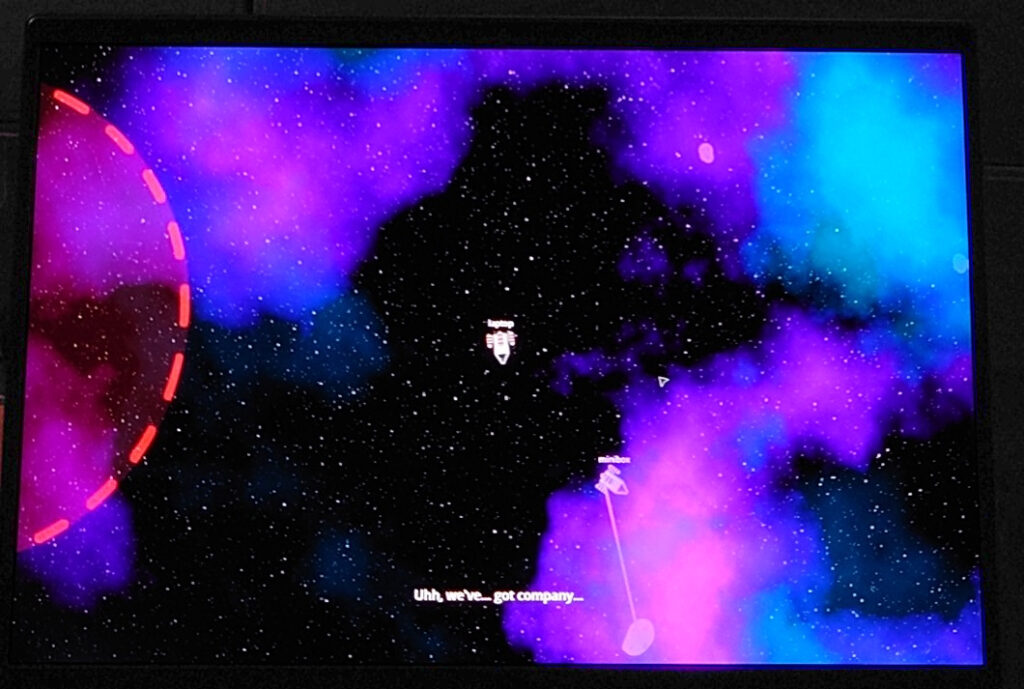
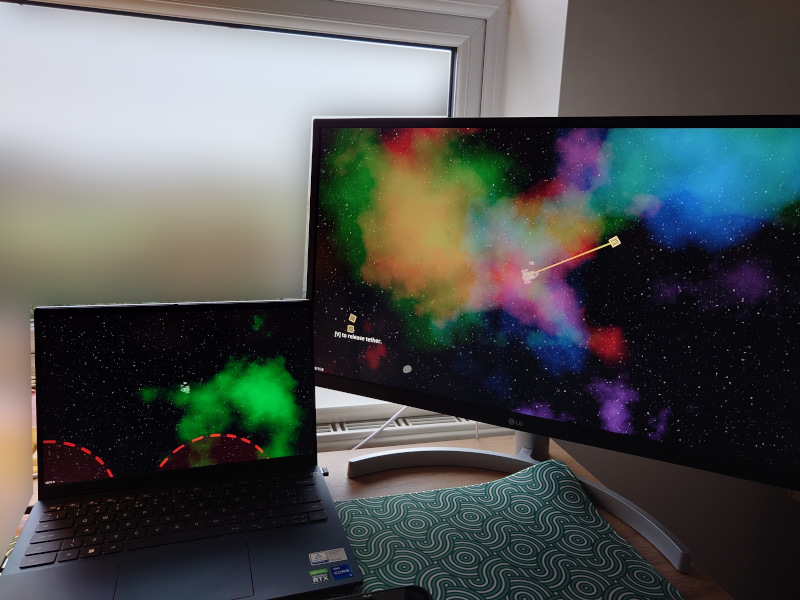
I had also previously tested spawning each nebula with completely random colouring, which often produced a complete mess.
I also added (and later removed) planet sprites into the game. I spent a bit too much time on these – including making shadows consistent per-sector. I later removed these small planets as I was considering adding larger planets that would appear small in early sectors, and slowly grow as if you were getting closer with each sector. These larger planets have not yet made it into release as I’ve still been toying with trying to make them as shaders instead of textures (to save on file sizes).
Audio
Music
I wrote Slingshottr’s music in FL Studio. I aimed for this music to be fairly ambient and not too intrusive. Moreover I didn’t want it to undermine the player’s experience in different situations.
For example, if the player is involved in an action-packed pirate fight, you probably wouldn’t want gentle piano music in the background. Similarly, 140bpm drum and bass might not fit so well with casual exploration.
My solution here was to write sci-fi-esque music that was slightly more on the gentle side, but still had some crescendos. The music found in Terraria, Minecraft, and Avorion were inspirations on this front.
“Song 5” from Slingshottr – still has some crescendo without being too intrusive.
There were also some scenarios where I wanted the music to change or stop. Notably during the Quasar Burst and Ghost Ship events the music will stop. (Eerie music begins in the latter event.)
To achieve the behaviour I wanted, (continuous play of tracks, interruptible by events), I wrote a Music manager class.
This class is very basic. If the player is in game, and the AudioStreamPlayer is not playing, pick a track and play it. I also decided to have the class keep note of the last played track so as not to play the same one twice in a row.
I also wrote a couple of small RPC methods that can be called over the network, for example when the server wants to tell all clients to stop or resume their music.
There are improvements that could be made to this system. For example, right now I’m checking if a track is finished every frame. Although the actual performance impact of this is negligible, it’s really not good practice and is the sort of bad habit that can add up. A simple fix here would be to use signals instead. AudioStreamPlayer has a finished() signal. When this signal is emitted, we could then run the ‘pick track’ method and play the chosen track. It would be important however to watch out for scenarios where other code might have relied on the music manager automatically picking and playing a track if nothing was being played.
Sound FX
Due to the nature of solo development, I decided it would be worthwhile to save some time and not try to create all the sound effects I needed. I used freesound.org for some sound effects, the full details and licenses of which can be found in the game’s credits.
Most sound effects I made in FL Studio, however there are a few fun exceptions.
The repair sound effects – when players are damaged and go in range of the repair station – were made by recording the sound of a handheld electric drill.
The Ghost Ship’s movement sounds were created by just recording my own breaths and adding a bit of reverb to make them more ‘ghostly’.
The Quasar Burst’s warning alarm sound I created by having two synth tones playing at the same time repeatedly. My inspiration was basically trying to replicate the sort of sounds commonly heard in real-world emergency broadcast systems.
Playtesting
Playtesting began very early on in the development cycle. Initially it was just me testing single player (and asking whoever was near me at the time to try it!)
As I started getting multiplayer working, I began sending copies to friends and organising regular weekly multiplayer playtests.
These tests were invaluable in finding bugs, refining features, and guiding development.
It was also very useful to learn how players were discovering what they could and couldn’t do in game. A great example of this is from a local multiplayer playtest I did in February 2024, wherein three friends (two who’d never played) played together.
One suggests the two players tether both some scrap and each other, while the more experienced player points out “You’ve only got one tether.” I’m not sure whether the first player meant both players should tether one piece of scrap (doable) or literally each tether a separate piece and eachother (pointless.)
This playtest also uncovered a new (albeit fun according to the testers) bug. At higher level sectors, (where difficulty had increased a lot), if the players did not complete the sector quickly enough they would become overrun with pirates. I patched this issue, but the later addition of limited ships would also have acted as a kind of solution.
In late March 2024 I made the playtest public on Steam. I made it open to anyone, and pushed updates weekly.